A while ago I explained my scripts (JSP and PHP) to handle RTF files, now I will show my built packages (Java and PHP) that include how to handle RTF, HTML, CSS and Script Files basically.
First you need to obtain my packages (Java or PHP)
bz-htmlcss2rtf.zip This zip file contains bz-htmlcss2rtf.jar and bz-htmlcss2rtf.phar and files used in this example.
The Java package can be used with Servlet or JSP scripts, my example for simplicity purpose will be in JSP scripts, you can to adapt it to Servlet.
I made two scripts to show you my examples both in JSP and PHP languages.
First Code – DBPer.xxp
DBPer.jsp
<%@page import="java.sql.*"%><%@page import="static bz.htmlcss2rtf.Ini.*"%>
<%@page import="bz.htmlcss2rtf.*"%><%@page errorPage ="error.jsp"%><%
try {
setPageContext(pageContext);
String webFile = getNameScript();
CSS CSSFile = new CSS("CSS0"); //Name
CSSFile.addInputStyle("Boton"," background-color:rgb(255,255,255); color:rgb(0,0,0);"
+" cursor:pointer; width:150px; padding:1px; border-width:4px;"
+" border-color:rgb(127,127,127); border-style:double; margin:1px; font-size: 8pt;"
+" font-family: Courier;");//Boton
CSSFile.addInputPseudoStyle("Boton","hover"," background-color:rgb(0,0,0);"
+" color:rgb(255,255,255); font-weight:bold;");
CSSFile.addInputPseudoStyle("Boton","disabled"," background-color:rgb(200,200,200);"
+" color:rgb(64,64,64);");
CSSFile.addDivStyle("Mssg"," background-color:rgb(220,220,220); position:absolute;"
+" padding-top: 4px; padding-bottom: 4px; padding-left: 4px; border-style:double;"
+" font-size:13px; text-shadow: rgb(255,255,255) 2px 2px; Width:37%; left:62%;"
+" top:2%; z-index:1;");//Mssg
CSSFile.addBodyStyle("Bod1","color:#7F7F7F;");
HTMLFile MsgFl = new HTMLFile("MsgFl","Messages","0"); //Name,[Title],[Charset_#]
MsgFl.setCSSObject(CSSFile);
MsgFl.setShortCutIcon("BZ.ico");
String StrMessg = "";
String mHost = "192.168.97.21";
String mUser = "root";
String mPass = "MyPassword";
String newHost = "%"; // or "localhost";
String newUser = "dbUser";
String newPass = "dbKey";
String newDB = "dbCompany";
setSessionObj("newUser",newUser);
setSessionObj("newPass",newPass);
setSessionObj("newDB",newDB);
String FindDB = "SELECT SCHEMA_NAME FROM INFORMATION_SCHEMA.SCHEMATA"
+" WHERE SCHEMA_NAME = '"+newDB+"';";
String CreateDB = "CREATE DATABASE "+newDB+";";
String DeleteDB = "DROP DATABASE IF EXISTS "+newDB+";";
String UseDB = "USE "+newDB+";";
String CreateTbl = "CREATE TABLE tblRecords (FirsLastName varchar(30),"
+"Salary int(11),JoinDate DATE,Photo MEDIUMBLOB,Resume MEDIUMTEXT);";
String CreateUser = "CREATE USER '";
String DropUser = "DROP USER '";
String GrantAll = "GRANT ALL ON ";
String Atsign = "'@'";
String Identified = "' IDENTIFIED BY '";
String FlushPriv = "FLUSH PRIVILEGES;";
String CreatingUser = CreateUser+newUser+Atsign+newHost+Identified+newPass+"';";
String GrantOverDB = GrantAll+newDB+".* TO '"+newUser+Atsign+newHost+"';";
Connection conn = newMySQLConn(mHost,mUser,mPass); //Host,User,Pass,[DB],[Port]
setConnection(conn);
ResultSet result = doQry(FindDB); //Peek for Database
if (qtyQry(result) < 1) {
if(getRequestStr("bCrtDB") != null) {
try {
doQry(CreateDB);
doQry(UseDB);
doQry(CreateTbl);
doQry(CreatingUser);
doQry(GrantOverDB);
doQry(FlushPriv);
} catch (Exception e) {
StrMessg += "Error: "+e.getMessage();
}
StrMessg += "The '"+newDB+"' database has been created...<br>";
HTMLFile gtFl = new HTMLFile("gtFl","create","0"); //Name,[Title],[Charset_#]
gtFl.setCSSObject(CSSFile);
gtFl.setShortCutIcon("BZ.ico");
HTMLForm gtFr = new HTMLForm("Etiq","Form",HTMLForm.FORM_POSTMULTIPART,
"PersEng.jsp"); //Name,FormID,Method,[Action]
gtFr.setCSSObject(CSSFile);
gtFl.addScriptPiece("FisrtScript","text/javascript"); //Name,Type
gtFl.addScriptLine("FisrtScript","var myVar=\"hello\";"); //Name,Line
gtFl.addScriptLine("FisrtScript","function showAlert() { alert('My alert!'); }");
gtFr.addInput("bgtPE",HTMLForm.INPUT_SUBMIT,"PersEng.jsp",
"Boton",""); //Name,Type,Value(TextToShow),Style,Argument0,[Argument1]
String insertButton = gtFr.getInput("bgtPE"); //Name,[...Events]
gtFr.addElement(insertButton); //insert the button to the Form
String insertForm = gtFr.getForm();
gtFl.addElement(insertForm); //insert the form to the File
String insertMessage = gtFl.getNewDiv(StrMessg,"Mssg"); //Content,[Style]
gtFl.addElement(insertMessage);
gtFl.closeFile();
String insertFile = gtFl.getFile("Bod1"); //[Style]
printSng(insertFile); //PrintSingle(TextToShow)
} else {
HTMLFile CrtFl = new HTMLFile("CreateDBFile","create","0");
CrtFl.setCSSObject(CSSFile);
CrtFl.setShortCutIcon("BZ.ico");
HTMLForm CrtFr = new HTMLForm("Etiq","Form",
HTMLForm.FORM_POST); //Name,FormID,Method,[Action]
CrtFr.setCSSObject(CSSFile);
CrtFr.addInput("bCrtDB",HTMLForm.INPUT_SUBMIT,"Create DB","Boton","");
CrtFr.addElement(CrtFr.getInput("bCrtDB"));
CrtFl.addElement(CrtFr.getForm());
CrtFl.addElement(CrtFl.getNewDiv(StrMessg,"Mssg"));
CrtFl.closeFile();
printSng(CrtFl.getFile("Bod1"));
}
} else {
if(getRequestStr("bDelDB") != null) {
StrMessg = "";
String DropAdmins = DropUser+newUser+Atsign+newHost+"';";
doQry(DropAdmins);
int RDropDB = doQry(DeleteDB);
if(RDropDB != 0) {
StrMessg += "The '"+newDB+"' database has been deleted...<br>";
MsgFl.resetFile();
MsgFl.addElement(MsgFl.getNewDiv(StrMessg,"Mssg"));
MsgFl.closeFile();
printSng(MsgFl.getFile("Bod1"));
}
} else {
HTMLFile DelFl = new HTMLFile("UserFile","delete","0");
DelFl.setCSSObject(CSSFile);
DelFl.setShortCutIcon("BZ.ico");
HTMLForm DelFr = new HTMLForm("Etiq","Form",HTMLForm.FORM_POST);
DelFr.setCSSObject(CSSFile);
DelFr.addInput("bDelDB",HTMLForm.INPUT_SUBMIT,"Borrar DB","Boton","");
DelFr.addElement(DelFr.getInput("bDelDB"));
DelFr.addInput("bgtPE",HTMLForm.INPUT_SUBMIT,"PersEng.jsp","Boton","");
DelFr.addFormEvent("bgtPE","PersEng","onClick","PersEng.jsp");
DelFr.addElement(DelFr.getInput("bgtPE","PersEng"));
DelFl.addElement(DelFr.getForm());
DelFl.addElement(DelFl.getNewDiv(StrMessg,"Mssg"));
DelFl.closeFile();
printSng(DelFl.getFile("Bod1"));
}
}
result.close();
conn.close();
}
catch (Exception e) {
printErr(e.getMessage());
}
%>
DBPer.php
<?php
namespace bz\htmlcss2rtf;
require_once("phar://bz-htmlcss2rtf.phar/HTMLFile.php");
require_once("phar://bz-htmlcss2rtf.phar/HTMLTable.php");
require_once("phar://bz-htmlcss2rtf.phar/HTMLForm.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFFile.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFTable.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFImage.php");
require_once("phar://bz-htmlcss2rtf.phar/Ini.php");
require_once("phar://bz-htmlcss2rtf.phar/CSS.php");
require_once("error.php");
use Exception;
try {
$WebFile = getNameScript();
$CSSFile = new CSS("CSS0"); //Name
$CSSFile->addInputStyle("Boton"," background-color:rgb(255,255,255); color:rgb(0,0,0);"
." cursor:pointer; width:150px; padding:1px; border-width:4px;"
." border-color:rgb(127,127,127); border-style:double; margin:1px; font-size: 8pt;"
." font-family: Courier;");//Boton
$CSSFile->addInputPseudoStyle("Boton","hover"," background-color:rgb(0,0,0);"
." color:rgb(255,255,255); font-weight:bold;");
$CSSFile->addInputPseudoStyle("Boton","disabled"," background-color:rgb(200,200,200);"
." color:rgb(64,64,64);");
$CSSFile->addDivStyle("Mssg"," background-color:rgb(220,220,220); position:absolute;"
." padding-top: 4px; padding-bottom: 4px; padding-left: 4px; border-style:double;"
." font-size:13px; text-shadow: rgb(255,255,255) 2px 2px; Width:37%; left:62%;"
." top:2%; z-index:1;");//Mssg
$CSSFile->addBodyStyle("Bod1","color:#7F7F7F;");
$MsgFl = new HTMLFile("MsgFl","Messages","0"); //Name,[Title],[Charset_#]
$MsgFl->setCSSObject($CSSFile);
$MsgFl->setShortCutIcon("BZ.ico");
$StrMessg = "";
$mHost = "192.168.97.21";
$mUser = "root";
$mPass = "MyPassword";
$newHost = "%"; // or "localhost";
$newUser = "dbUser";
$newPass = "dbKey";
$newDB = "dbCompany";
session_start();
setSessionObj("newUser",$newUser);
setSessionObj("newPass",$newPass);
setSessionObj("newDB",$newDB);
$FindDB = "SELECT SCHEMA_NAME FROM INFORMATION_SCHEMA.SCHEMATA"
." WHERE SCHEMA_NAME = '".$newDB."';";
$CreateDB = "CREATE DATABASE ".$newDB.";";
$DeleteDB = "DROP DATABASE IF EXISTS ".$newDB.";";
$UseDB = "USE ".$newDB.";";
$CreateTbl = "CREATE TABLE tblRecords (FirsLastName varchar(30),"
."Salary int(11),JoinDate DATE,Photo MEDIUMBLOB,Resume MEDIUMTEXT);";
$CreateUser = "CREATE USER '";
$DropUser = "DROP USER '";
$GrantAll = "GRANT ALL ON ";
$Atsign = "'@'";
$Identified = "' IDENTIFIED BY '";
$FlushPriv = "FLUSH PRIVILEGES;";
$CreatingUser = $CreateUser.$newUser.$Atsign.$newHost.$Identified.$newPass."';";
$GrantOverDB = $GrantAll.$newDB.".* TO '".$newUser.$Atsign.$newHost."';";
$conn = newMySQLConn($mHost,$mUser,$mPass); //Host,User,Pass,[DB],[Port]
setConnection($conn);
$result = doQry($FindDB); //Peek for Database
if (qtyQry($result) < 1) {
if(getRequestStr("bCrtDB") != NULL) {
try {
doQry($CreateDB);
doQry($UseDB);
doQry($CreateTbl);
doQry($CreatingUser);
doQry($GrantOverDB);
doQry($FlushPriv);
} catch (Exception $e) {
$StrMessg .= "Error: ".$e.getMessage();
}
$StrMessg .= "The '".$newDB."' database has been created...<br>";
$gtFl = new HTMLFile("gtFl","create","0"); //Name,[Title],[Charset_#]
$gtFl->setCSSObject($CSSFile);
$gtFl->setShortCutIcon("BZ.ico");
$gtFr = new HTMLForm("Etiq","Form",HTMLForm::FORM_POSTMULTIPART,
"PersEng.php"); //Name,FormID,Method,[Action]
$gtFr->setCSSObject($CSSFile);
$gtFl->addScriptPiece("FisrtScript","text/javascript"); //Name,Type
$gtFl->addScriptLine("FisrtScript","var myVar=\"hello\";"); //Name,Line
$gtFl->addScriptLine("FisrtScript","function showAlert() { alert('My alert!'); }");
$gtFr->addInput("bgtPE",HTMLForm::INPUT_SUBMIT,"PersEng.php",
"Boton",""); //Name,Type,Value(TextToShow),Style,Argument0,[Argument1]
$insertButton = $gtFr->getInput("bgtPE"); //Name,[...Events]
$gtFr->addElement($insertButton); //insert the button to the Form
$insertForm = $gtFr->getForm();
$gtFl->addElement($insertForm); //insert the form to the File
$insertMessage = $gtFl->getNewDiv($StrMessg,"Mssg"); //Content,[Style]
$gtFl->addElement($insertMessage);
$gtFl->closeFile();
$insertFile = $gtFl->getFile("Bod1"); //[Style]
printSng($insertFile); //PrintSingle(TextToShow)
} else {
$CrtFl = new HTMLFile("CreateDBFile","create","0");
$CrtFl->setCSSObject($CSSFile);
$CrtFl->setShortCutIcon("BZ.ico");
$CrtFr = new HTMLForm("Etiq","Form",
HTMLForm::FORM_POST); //Name,FormID,Method,[Action]
$CrtFr->setCSSObject($CSSFile);
$CrtFr->addInput("bCrtDB",HTMLForm::INPUT_SUBMIT,"Create DB","Boton","");
$CrtFr->addElement($CrtFr->getInput("bCrtDB"));
$CrtFl->addElement($CrtFr->getForm());
$CrtFl->addElement($CrtFl->getNewDiv($StrMessg,"Mssg"));
$CrtFl->closeFile();
printSng($CrtFl->getFile("Bod1"));
}
} else {
if(getRequestStr("bDelDB") != NULL) {
$StrMessg = "";
$DropAdmins = $DropUser.$newUser.$Atsign.$newHost."';";
doQry($DropAdmins);
$RDropDB = doQry($DeleteDB);
if($RDropDB != 0) {
$StrMessg .= "The '".$newDB."' database has been deleted...<br>";
$MsgFl->resetFile();
$MsgFl->addElement($MsgFl->getNewDiv($StrMessg,"Mssg"));
$MsgFl->closeFile();
printSng($MsgFl->getFile("Bod1"));
}
} else {
$DelFl = new HTMLFile("UserFile","delete","0");
$DelFl->setCSSObject($CSSFile);
$DelFl->setShortCutIcon("BZ.ico");
$DelFr = new HTMLForm("Etiq","Form",HTMLForm::FORM_POST);
$DelFr->setCSSObject($CSSFile);
$DelFr->addInput("bDelDB",HTMLForm::INPUT_SUBMIT,"Borrar DB","Boton","");
$DelFr->addElement($DelFr->getInput("bDelDB"));
$DelFr->addInput("bgtPE",HTMLForm::INPUT_SUBMIT,"PersEng.php","Boton","");
$DelFr->addFormEvent("bgtPE","PersEng","onClick","PersEng.php");
$DelFr->addElement($DelFr->getInput("bgtPE","PersEng"));
$DelFl->addElement($DelFr->getForm());
$DelFl->addElement($DelFl->getNewDiv($StrMessg,"Mssg"));
$DelFl->closeFile();
printSng($DelFl->getFile("Bod1"));
}
}
$result->close();
$conn->close();
}
catch (Exception $e) {
printErr($e->getMessage().":".$e->getFile().":".$e->getLine());
}
?>
This code displays four pages, depending on the status of a MySQL database if not exists then create it, if there is then given the opportunity to operate it or delete it.
When DbCompany not exist.
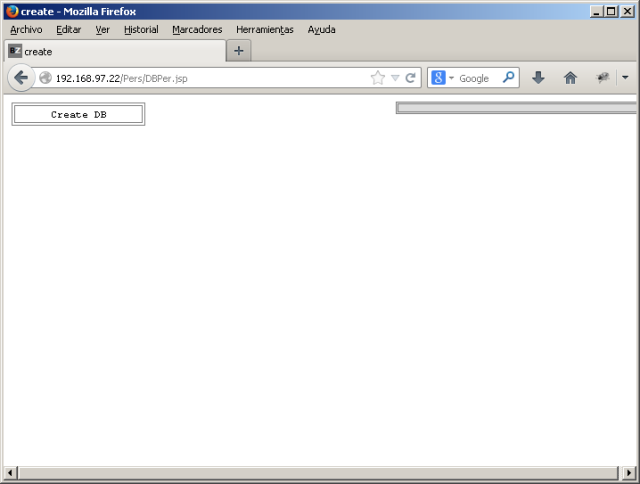
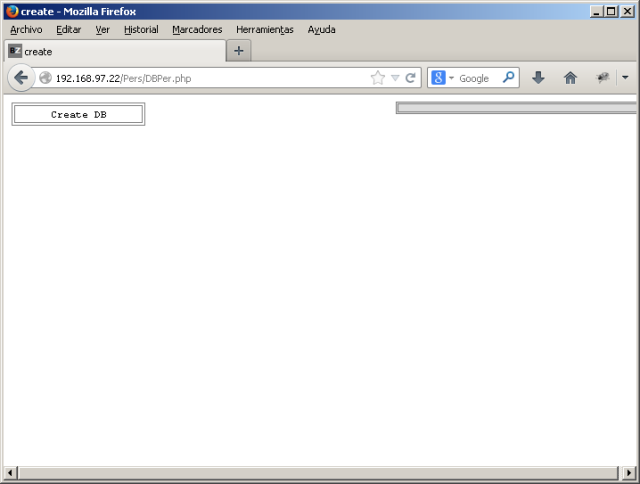
When DbCompany created.
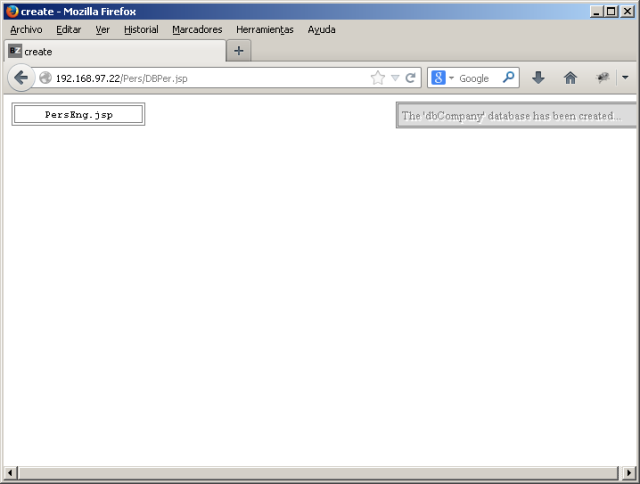
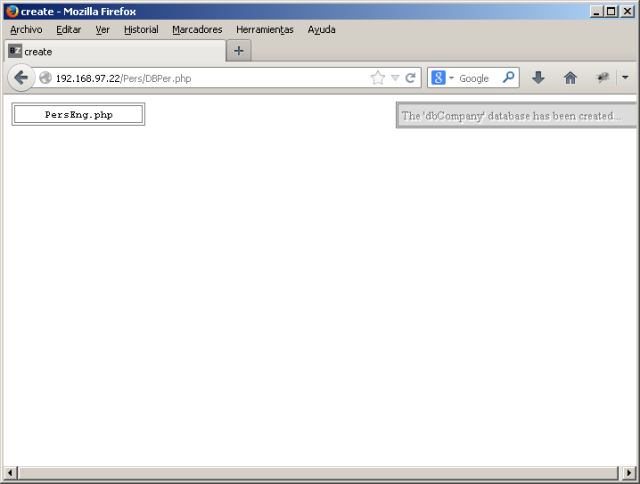
When DbCompany there may be operated or removed.
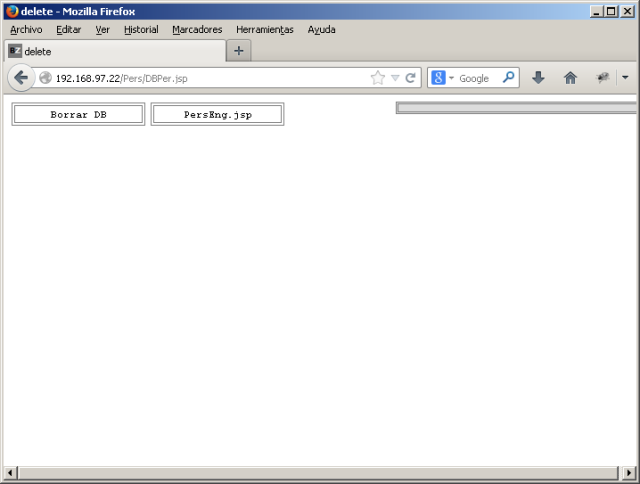
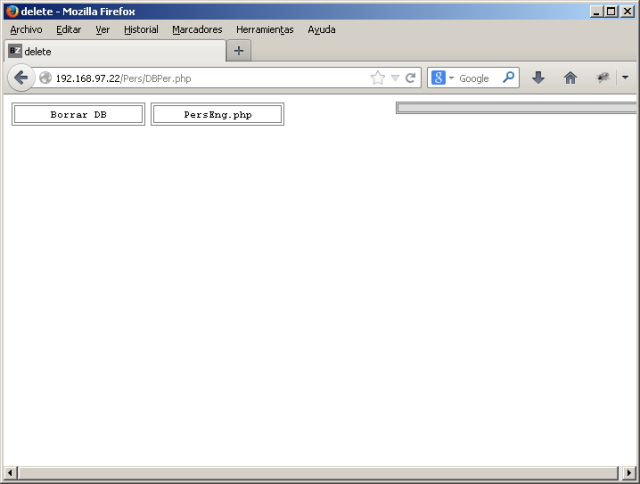
When DbCompany has been removed.
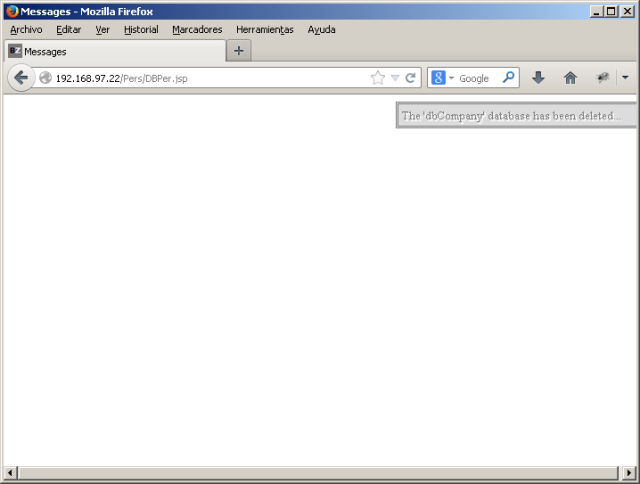
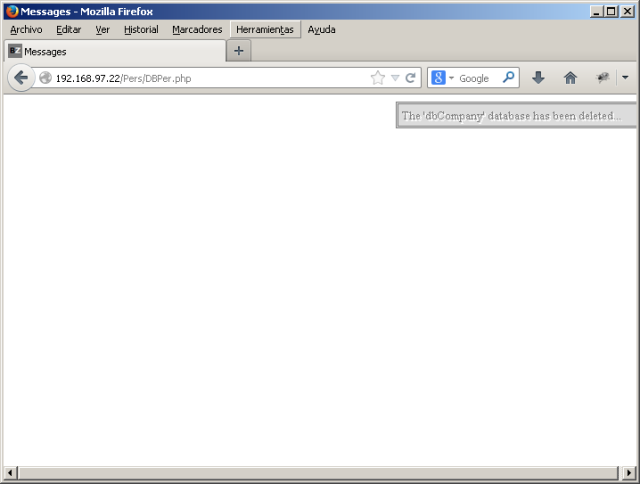
Second Code – PersEng.xxp
PersEng.jsp
<%@page import="static bz.htmlcss2rtf.Ini.*"%><%@page import="bz.htmlcss2rtf.*"%>
<%@page import="java.sql.*"%><%@page import="java.io.*"%><%@page errorPage ="error.jsp"%><%
try {
setPageContext(pageContext);
String WebPath = getPathScript();
String WebRoot = getRootService()+WebPath;
String FS = separatorFileDir();//Separador de Archivo o de Directorio
String ScriptRoot = getPathService(WebPath);
CSS MainCSS = new CSS("MainCSS");
HTMLFile mFile = new HTMLFile("mFile",getStrictName(),"0"); //Name,[Title],[Charset_#]
mFile.setShortCutIcon("BZ.ico");
mFile.setCSSObject(MainCSS);
mFile.addCSSFile("Pers.css");
HTMLForm MainForm = new HTMLForm("MainForm","Form",
HTMLForm.FORM_POSTMULTIPART); //Name,FormID,Method,[Action]
MainForm.setCSSObject(MainCSS);
MainForm.addInput("tName",HTMLForm.INPUT_TEXT,"","Texto",
"30"); //Name,Type,Value(empty),Style,Argument0(size),[Argument1]
MainForm.addInput("tSalary",HTMLForm.INPUT_TEXT,"","Texto","11");
MainForm.addInput("tJoinDate",HTMLForm.INPUT_TEXT,"","Texto","10");
MainForm.addInput("fPhoto",HTMLForm.INPUT_FILE,"","File","60");
MainForm.addInput("fResume",HTMLForm.INPUT_FILE,"","File","60");
MainForm.addInput("bLoad",HTMLForm.INPUT_SUBMIT,"Load Data","Boton","");
MainForm.addInput("bList",HTMLForm.INPUT_SUBMIT,"List Data","Boton","");
MainForm.addInput("bDelt",HTMLForm.INPUT_SUBMIT,"Delete Data","Boton","");
MainForm.addInput("bRstJ",HTMLForm.INPUT_SUBMIT,"JSP Home","Boton","");
MainForm.addInput("bRstP",HTMLForm.INPUT_SUBMIT,"PHP Home","Boton","");
MainForm.addFormEvent("bLoad","jsp","onClick",
getNameScript()); //Owner,IntID(InternalID),Event,Action
MainForm.addInputEvent("bLoad","jsp2","onClick2",
"Pers2.jsp"); //Owner,IntID(InternalID),Event,Action
MainForm.addFormEvent("bLoad","php","onClick",getStrictName()+".php");
MainForm.addFormEvent("bList","jsp","onClick",getNameScript());
MainForm.addFormEvent("bList","php","onClick",getStrictName()+".php");
MainForm.addFormEvent("bDelt","jsp","onClick",getNameScript());
MainForm.addFormEvent("bDelt","php","onClick",getStrictName()+".php");
MainForm.addFormEvent("bRstJ","jsp","onClick",getNameScript());
MainForm.addFormEvent("bRstP","php","onClick",getStrictName()+".php");
HTMLTable MainTabl = new HTMLTable("MainTabl",2,4); //Name,NumCols,NumRows
MainTabl.setCSSObject(MainCSS);
MainTabl.setHeadCol(0,"Data Records");
MainTabl.mergeHeadCol(0,2); //Col,Value(# TobeMerged)
MainTabl.mergeHorzCell(0,0,2);
MainTabl.setTextCell(0,0,MainForm.getLabelInp("First and Last Names:",
"tName","Etiq")+MainForm.getInput("tName"));
MainTabl.setTextCell(0,1,MainForm.getLabelInp("Salary Value:","tSalary",
"Etiq")+MainForm.getInput("tSalary"));
MainTabl.setTextCell(1,1,MainForm.getLabelInp("Join Date:","tJoinDate",
"Etiq")+MainForm.getInput("tJoinDate"));
MainTabl.mergeHorzCell(0,2,2);
MainTabl.setTextCell(0,2,MainForm.getLabelInp("Recent photo:","fPhoto",
"Etiq")+MainForm.getInput("fPhoto"));
MainTabl.mergeHorzCell(0,3,2);
MainTabl.setTextCell(0,3,MainForm.getLabelInp("Resume:","fResume",
"Etiq")+MainForm.getInput("fResume"));
HTMLTable ButtTabl = new HTMLTable("ButtTabl",4,2);
ButtTabl.setCSSObject(MainCSS);
ButtTabl.setHeadRow(0,getNameScript());
ButtTabl.setHeadRow(1,getStrictName()+".php");
ButtTabl.setTextCell(0,0,MainForm.getInput("bLoad","jsp",
"jsp2")); //Name,[...Events](2 Events)
ButtTabl.setTextCell(1,0,MainForm.getInput("bList","jsp"));
ButtTabl.setTextCell(2,0,MainForm.getInput("bDelt","jsp"));
ButtTabl.setTextCell(3,0,MainForm.getInput("bRstJ","jsp"));
ButtTabl.setTextCell(0,1,MainForm.getInput("bLoad","php"));
ButtTabl.setTextCell(1,1,MainForm.getInput("bList","php"));
ButtTabl.setTextCell(2,1,MainForm.getInput("bDelt","php"));
ButtTabl.setTextCell(3,1,MainForm.getInput("bRstP","php"));
MainForm.addElement(MainTabl.getTable());
MainForm.addElement(ButtTabl.getTable());
mFile.addElement(MainForm.getForm());
mFile.closeFile();
printSng(mFile.getFile());
//MySQL Connection
String host = "192.168.97.21"; //"192.168.97.21:3306"; //"localhost";
String port = "3306";
String OldUser = "dbUserf";
String OldPass = "dbKeyf";
String OldDB = "dbCompanyf";
if (getSessionStr("newUser") != null) {
OldUser = getSessionStr("newUser");
}
if (getSessionStr("newPass") != null) {
OldPass = getSessionStr("newPass");
}
if (getSessionStr("newDB") != null) {
OldDB = getSessionStr("newDB");
}
Connection newConn = null;
ResultSet rsPE = null;
PreparedStatement PE = null;
newConn = newMySQLConn(host,OldUser,OldPass,OldDB,
port); //Host,User,Password,[DataBase],[Port]
setConnection(newConn);
//Insertion
if (getRequestStr("bLoad")!=null) {
try {
PE = newConn.prepareStatement("INSERT INTO tblRecords (FirsLastName,"
+"Salary,JoinDate,Photo,Resume) VALUES(?,?,?,?,?)");
String sName = getRequestStr("tName");
String sSalary = getRequestStr("tSalary");
String sJoinDate = getRequestStr("tJoinDate");
InputStream isPhoto = getRequestFileStream("fPhoto");
String sResume = getRequestFileString("fResume");
PE.setString(1,sName);
PE.setInt(2,Integer.parseInt(sSalary));
PE.setString(3,sJoinDate);
PE.setBinaryStream(4,isPhoto);
PE.setString(5,sResume);
PE.executeUpdate();
printMsg("Successful insertion!");
}
catch (Exception e) { printErr(e.toString()); }
finally {
PE.close();
newConn.close();
}
}
//Extraction
if (getRequestStr("bList")!=null) {
String FileName = "Personal.JSP.RTF";
String FullFileName = ScriptRoot+FileName;
String WebFileName = WebRoot+FileName;
String sPathJPG = ScriptRoot+"joseluisbz.jpg";
String sPathPNG = ScriptRoot+"Empresa.png";
try {
BufferedWriter bw = new BufferedWriter(new FileWriter(FullFileName));
RTFFile FileRTF = new RTFFile();
RTFImage imgJPG = new RTFImage(sPathJPG);
if (imgJPG.getHexImage().equals("Error")) {
throw new RuntimeException("Error: File \""+sPathJPG+"\" is not JPG or PNG");
}
/*
//predefined Colors that do not need to be added
//Name,Red,Green,Blue
FileRTF.addColor("Negro",0,0,0); //Black ->Index(0)
FileRTF.addColor("Azul",0,0,255); //Blue ->Index(1)
FileRTF.addColor("Cian",0,255,255); //Cyan ->Index(2)
FileRTF.addColor("Verde",0,255,0); //Green ->Index(3)
FileRTF.addColor("Magenta",255,0,255); //Magenta ->Index(4)
FileRTF.addColor("Rojo",255,0,0); //Red ->Index(5)
FileRTF.addColor("Amarillo",255,255,0); //Yellow ->Index(6)
FileRTF.addColor("Blanco",255,255,255); //White ->Index(7)
//predefined Fonts that do not need to be added
//Name,Family,FamilyName
FileRTF.addFont("TlwgMono","fnil","TlwgMono");
FileRTF.addFont("Arial","fswiss","Arial");
FileRTF.addFont("TimesNewRoman","froman","Times New Roman");
FileRTF.addFont("CourierNew","fmodern","Courier New");
FileRTF.addFont("Edwardian","fscript","Edwardian Script ITC");
*/
String InfImagenJPG = imgJPG.getRTFImage(imgJPG.getHexImage(),imgJPG.getFormat(),
imgJPG.getHigh(),imgJPG.getWide());
RTFImage imgPNG = new RTFImage(sPathPNG);
if (imgPNG.getHexImage().equals("Error")) {
throw new RuntimeException("Error: File \""+sPathPNG+"\" is not JPG or PNG");
}
String InfImagenPNG = imgPNG.getRTFImage(imgPNG.getHexImage(),imgPNG.getFormat(),
imgPNG.getHigh(),imgPNG.getWide());
RTFImage ImageRTFIMG;
String InfImagenIMG;
PE = newConn.prepareStatement("SELECT * FROM tblRecords");
rsPE = PE.executeQuery();
rsPE.last();
int NumRecords = rsPE.getRow();rsPE.beforeFirst();
RTFTable TblData = null;
FileRTF.addList("Lst1",RTFFile.LIST_UPPERROMANS,"1"); //Name,Type,FamilyFont#
FileRTF.addListItem("Lst1","Primero");
FileRTF.addListItem("Lst1","Segundo");
FileRTF.addList("Lst2",RTFFile.LIST_LOWERALPHABET,"2");
FileRTF.addListItem("Lst2","First");
FileRTF.addListItem("Lst2","Second");
while(rsPE.next()) {
FileRTF.offOtherFormatFile();
FileRTF.setAlign(RTFFile.ALIGN_CENTERED);
FileRTF.setColorFront("Blanco");
FileRTF.setColorBack("Negro");
FileRTF.setFont("Edwardian");
FileRTF.setSizeFont(20);
FileRTF.setUnderLine(RTFFile.ON);
FileRTF.addText("Limited Company");
FileRTF.addParg();
FileRTF.setColorBack("Blanco");
FileRTF.setColorFront("Negro");
TblData = new RTFTable(4,4); //4 Columns, 4 Rows
TblData.setTableSpaceCellsRow(10);
TblData.setTableLeftmostEdgePos(10);
TblData.setTableHorzAlign(RTFTable.TABLE_LEFT);
TblData.setTableBorderType(RTFTable.BORDER_SINGLETHICKNESS);
TblData.setCellsVertAlign(RTFTable.CELLS_TOP);
TblData.setCellsBorderType(RTFTable.BORDER_DOUBLETHICKNESS);
TblData.setCellsThick(10);
TblData.setCellsColor(FileRTF.getColorIndex("Negro"));
TblData.setWideCols(1000); //Width of 2000 to put all columns
TblData.setWideCol(0,3000); //Width of 3000 to Column 0
TblData.setWideCol(3,3000); //Width of 3000 to Column 3
TblData.setAlignTable(RTFTable.ALIGN_CENTERED);
TblData.setColorFrontTable(FileRTF.getColorIndex("Blanco"));
TblData.setColorBackTable(FileRTF.getColorIndex("Negro"));
TblData.setFontTable(FileRTF.getFontIndex("TlwgMono"));
TblData.setSizeFontTable(12);
TblData.setAlignCell(0,0,RTFTable.ALIGN_LEFT); //Col,Row,Align
TblData.setColorFrontCell(0,0,0); //Col,Row,ColorIndex
TblData.setColorBackCell(0,0,0); //Col,Row,ColorIndex
TblData.setFontCell(0,0,0); //Col,Row,Font
TblData.setSizeFontCell(0,0,12); //Col,Row,Size
TblData.setItemCell(0,1,InfImagenPNG);
TblData.setItemCell(3,1,InfImagenJPG);
TblData.mergeHorzCell(1,0,2); //Col,Row,NumCellToBeMergedHorizontally
TblData.setTextCell(1,0,"Celda 1,0 con Celda 2,0");
TblData.mergeHorzCell(1,3,2);
TblData.setTextCell(1,3,"Celda 1,3 con Celda 2,3");
TblData.mergeVertCell(0,1,2); //Col,Row,NumCellToBeMergedVerticaally
TblData.mergeVertCell(3,1,2);
FileRTF.addItem(TblData.getTable());
FileRTF.addLine();
FileRTF.setUnderLine(RTFFile.OFF);
FileRTF.setSizeFont(11);
FileRTF.setFont("CourierNew");
FileRTF.setScript(RTFFile.SUB);
FileRTF.addText("SUBSCRIPT");
FileRTF.setScript(RTFFile.OFF);
FileRTF.addText(" ");
FileRTF.setStrike(RTFFile.ON);
FileRTF.addText("STRIKE");
FileRTF.setStrike(RTFFile.OFF);
FileRTF.addText(" ");
FileRTF.setScript(RTFFile.SUPER);
FileRTF.addText("SUPERSCRIPT");
FileRTF.setScript(RTFFile.OFF);
FileRTF.addLine();
FileRTF.setAlign(RTFFile.ALIGN_LEFT);
FileRTF.setFont("CourierNew");
FileRTF.setSizeFont(12);
FileRTF.setUnderLine(RTFFile.ON);
FileRTF.setBold(RTFFile.OFF);
FileRTF.setItalic(RTFFile.OFF);
FileRTF.setColorFront("Negro");
FileRTF.addText("Nombre: ");
FileRTF.setItalic(RTFFile.ON);
FileRTF.setUnderLine(RTFFile.OFF);
FileRTF.setColorFront("Rojo");
FileRTF.addText(rsPE.getString(1));
FileRTF.addLine();
FileRTF.setItalic(RTFFile.OFF);
FileRTF.setUnderLine(RTFFile.ON);
FileRTF.setColorFront("Negro");
FileRTF.addText("Salario: ");
FileRTF.setItalic(RTFFile.ON);
FileRTF.setUnderLine(RTFFile.OFF);
FileRTF.setColorFront("Rojo");
FileRTF.addText(String.valueOf(rsPE.getInt(2)));
FileRTF.addLine();
ImageRTFIMG = new RTFImage(rsPE.getBytes(4));
/*
//ImageRTFIMG.setCropLeft(0);// 0 cutted twips in Left Side
//ImageRTFIMG.setCropRight(0);// 0 cutted twips in Right Side
//ImageRTFIMG.setCropTop(0);// 0 cutted twips in Top Side
//ImageRTFIMG.setCropBottom(0);// 0 cutted twips in Bootom Side
//ImageRTFIMG.setScaleX(100);// 100% of X Image
//ImageRTFIMG.setScaleY(100);// 100% of Y Image
//*/
ImageRTFIMG.setScaleX(50);
ImageRTFIMG.setScaleY(25);
InfImagenIMG = ImageRTFIMG.getRTFImage(ImageRTFIMG.getHexImage(),
ImageRTFIMG.getFormat(),ImageRTFIMG.getHigh(),ImageRTFIMG.getWide());
FileRTF.addItem(InfImagenIMG);
FileRTF.addParg(); //Separe Image of Text
FileRTF.setItalic(RTFFile.OFF);
FileRTF.setUnderLine(RTFFile.ON);
FileRTF.setColorFront("Negro");
FileRTF.addText("Resumen: ");
FileRTF.setItalic(RTFFile.ON);
FileRTF.setUnderLine(RTFFile.OFF);
FileRTF.setColorFront("Verde");
FileRTF.addText(rsPE.getString(5));
FileRTF.setColorFront("Azul");
FileRTF.addLine();
FileRTF.addText("Ini List");
FileRTF.setFirstIndent(-360);
FileRTF.setLeftIndent(720);
FileRTF.setSpaceAfter(RTFFile.ON);
FileRTF.setSpaceMultiple(RTFFile.ON);
FileRTF.addItem(FileRTF.getList("Lst1"));
FileRTF.offIndent();
FileRTF.offSpacing();
FileRTF.incIndent();
FileRTF.incSpacing();
FileRTF.addLine();
FileRTF.addText("Mid List");
FileRTF.setSpaceLine(RTFFile.SL_2_0); //Line Spacing 2.0
FileRTF.setRightIndent(1040);
FileRTF.setSpaceBefore(RTFFile.ON);
FileRTF.setSpaceMultiple(RTFFile.ON);
FileRTF.addItem(FileRTF.getList("Lst2"));
FileRTF.offIndent();
FileRTF.offSpacing();
FileRTF.incIndent();
FileRTF.incSpacing();
FileRTF.setColorFront("Negro");
FileRTF.addText("End List");
FileRTF.addLine();
FileRTF.addText("Record: "+NumRecords);
FileRTF.addLine();
FileRTF.addText("Last Text");
NumRecords--;
if (NumRecords>0) {
FileRTF.addParg();
FileRTF.addPage(); //New Page
}
}
FileRTF.endFile();
bw.write(FileRTF.getFile());
bw.close();
printLnk(WebFileName);
printMsg("Successful generation of RTF file!");
}
catch (Exception e) { printErr(e.toString()); }
finally {
rsPE.close();
PE.close();
newConn.close();
}
}
//Elimination
if (getRequestStr("bDelt")!=null) {
try {
PE = newConn.prepareStatement("DELETE FROM tblRecords");
PE.executeUpdate();
printMsg("Successful elimination!");
}
catch (Exception e) { printErr(e.toString()); }
finally {
PE.close();
newConn.close();
}
}
}
catch (Exception e) { printErr(e.toString()); }
%>
PersEng.php
<?php
namespace bz\htmlcss2rtf;
require_once("phar://bz-htmlcss2rtf.phar/HTMLFile.php");
require_once("phar://bz-htmlcss2rtf.phar/HTMLTable.php");
require_once("phar://bz-htmlcss2rtf.phar/HTMLForm.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFFile.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFTable.php");
require_once("phar://bz-htmlcss2rtf.phar/RTFImage.php");
require_once("phar://bz-htmlcss2rtf.phar/Ini.php");
require_once("phar://bz-htmlcss2rtf.phar/CSS.php");
require_once("error.php");
use Exception;
try {
$WebPath = getPathScript();
$WebRoot = getRootService().$WebPath;
$FS = separatorFileDir();//Separador de Archivo o de Directorio
$ScriptRoot = getPathService($WebPath);
$MainCSS = new CSS("MainCSS");
$mFile = new HTMLFile("mFile",getStrictName(),"0"); //Name,[Title],[Charset_#]
$mFile->setShortCutIcon("BZ.ico");
$mFile->setCSSObject($MainCSS);
$mFile->addCSSFile("Pers.css");
$MainForm = new HTMLForm("MainForm","Form",
HTMLForm::FORM_POSTMULTIPART); //Name,FormID,Method,[Action]
$MainForm->setCSSObject($MainCSS);
$MainForm->addInput("tName",HTMLForm::INPUT_TEXT,"","Texto",
"30"); //Name,Type,Value(empty),Style,Argument0(size),[Argument1]
$MainForm->addInput("tSalary",HTMLForm::INPUT_TEXT,"","Texto","11");
$MainForm->addInput("tJoinDate",HTMLForm::INPUT_TEXT,"","Texto","10");
$MainForm->addInput("fPhoto",HTMLForm::INPUT_FILE,"","File","60");
$MainForm->addInput("fResume",HTMLForm::INPUT_FILE,"","File","60");
$MainForm->addInput("bLoad",HTMLForm::INPUT_SUBMIT,"Load Data","Boton","");
$MainForm->addInput("bList",HTMLForm::INPUT_SUBMIT,"List Data","Boton","");
$MainForm->addInput("bDelt",HTMLForm::INPUT_SUBMIT,"Delete Data","Boton","");
$MainForm->addInput("bRstP",HTMLForm::INPUT_SUBMIT,"PHP Home","Boton","");
$MainForm->addInput("bRstJ",HTMLForm::INPUT_SUBMIT,"JSP Home","Boton","");
$MainForm->addFormEvent("bLoad","php","onClick",
getNameScript()); //Owner,IntID(InternalID),Event,Action
$MainForm->addInputEvent("bLoad","php2","onClick2",
"Pers2.php"); //Owner,IntID(InternalID),Event,Action
$MainForm->addFormEvent("bLoad","jsp","onClick",getStrictName().".jsp");
$MainForm->addFormEvent("bList","php","onClick",getNameScript());
$MainForm->addFormEvent("bList","jsp","onClick",getStrictName().".jsp");
$MainForm->addFormEvent("bDelt","php","onClick",getNameScript());
$MainForm->addFormEvent("bDelt","jsp","onClick",getStrictName().".jsp");
$MainForm->addFormEvent("bRstP","php","onClick",getNameScript());
$MainForm->addFormEvent("bRstJ","jsp","onClick",getStrictName().".jsp");
$MainTabl = new HTMLTable("MainTabl",2,4); //Name,NumCols,NumRows
$MainTabl->setCSSObject($MainCSS);
$MainTabl->setHeadCol(0,"Data Records");
$MainTabl->mergeHeadCol(0,2); //Col,Value(# TobeMerged)
$MainTabl->mergeHorzCell(0,0,2);
$MainTabl->setTextCell(0,0,$MainForm->getLabelInp("First and Last Names:",
"tName","Etiq").$MainForm->getInput("tName"));
$MainTabl->setTextCell(0,1,$MainForm->getLabelInp("Salary Value:",
"tSalary","Etiq").$MainForm->getInput("tSalary"));
$MainTabl->setTextCell(1,1,$MainForm->getLabelInp("Join Date:","tJoinDate",
"Etiq").$MainForm->getInput("tJoinDate"));
$MainTabl->mergeHorzCell(0,2,2);
$MainTabl->setTextCell(0,2,$MainForm->getLabelInp("Recent photo:","fPhoto",
"Etiq").$MainForm->getInput("fPhoto"));
$MainTabl->mergeHorzCell(0,3,2);
$MainTabl->setTextCell(0,3,$MainForm->getLabelInp("Resume:","fResume",
"Etiq").$MainForm->getInput("fResume"));
$ButtTabl = new HTMLTable("ButtTabl",4,2);
$ButtTabl->setCSSObject($MainCSS);
$ButtTabl->setHeadRow(0,getNameScript());
$ButtTabl->setHeadRow(1,getStrictName().".jsp");
$ButtTabl->setTextCell(0,0,$MainForm->getInput("bLoad","php",
"php2")); //Name,[...Events](2 Events)
$ButtTabl->setTextCell(1,0,$MainForm->getInput("bList","php"));
$ButtTabl->setTextCell(2,0,$MainForm->getInput("bDelt","php"));
$ButtTabl->setTextCell(3,0,$MainForm->getInput("bRstP","php"));
$ButtTabl->setTextCell(0,1,$MainForm->getInput("bLoad","jsp"));
$ButtTabl->setTextCell(1,1,$MainForm->getInput("bList","jsp"));
$ButtTabl->setTextCell(2,1,$MainForm->getInput("bDelt","jsp"));
$ButtTabl->setTextCell(3,1,$MainForm->getInput("bRstJ","jsp"));
$MainForm->addElement($MainTabl->getTable());
$MainForm->addElement($ButtTabl->getTable());
$mFile->addElement($MainForm->getForm());
$mFile->closeFile();
printSng($mFile->getFile());
//MySQL Connection
$host = "192.168.97.21"; //"192.168.97.21:3306"; //"localhost";
$port = "3306";
$OldUser = "dbUserf";
$OldPass = "dbKeyf";
$OldDB = "dbCompanyf";
session_start();
if (getSessionObj("newUser") != NULL) {
$OldUser = getSessionObj("newUser");
}
if (getSessionObj("newPass") != NULL) {
$OldPass = getSessionObj("newPass");
}
if (getSessionObj("newDB") != NULL) {
$OldDB = getSessionObj("newDB");
}
$newConn = NULL;
$PE = NULL;
$newConn = newMySQLConn($host,$OldUser,$OldPass,$OldDB,
$port); //Host,User,Password,[DataBase],[Port]
setConnection($newConn);
//Insertion
if (getRequestStr("bLoad")!= NULL) {
try {
$PE = $newConn->prepare("INSERT INTO tblRecords (FirsLastName,"
."Salary,JoinDate,Photo,Resume) VALUES(?,?,?,?,?)");
if (!$PE) {
throw new RuntimeException("Prepare SQL statement for creation failed!");
}
$sName = utf8_encode(getRequestStr("tName"));
$sSalary = getRequestStr("tSalary");
$sJoinDate = getRequestStr("tJoinDate");
$isPhoto = getRequestFileString("fPhoto");
$sResume = utf8_encode(getRequestFileString("fResume"));
$PE->bind_param('sisbb',$sName,$sSalary,$sJoinDate,$isPhoto,$sResume);
$PE->send_long_data(3,$isPhoto);
$PE->send_long_data(4,$sResume);
if (!$PE->execute()) {
throw new RuntimeException("Prepare SQL statement for execution failed!");
}
printMsg("Successful insertion!");
}
catch (Exception $e) { printErr($e->getMessage()); }
finally {
$PE->close();
$newConn->close();
}
}
//Extraction
if (getRequestStr("bList")!= NULL) {
$FileName = "Personal.PHP.RTF";
$FullFileName = $ScriptRoot.$FileName;
$WebFileName = $WebRoot.$FileName;
$sPathJPG = $ScriptRoot."joseluisbz.jpg";
$sPathPNG = $ScriptRoot."Empresa.png";
try {
$fw = fopen($FullFileName,"wb");
if (!$fw) {
throw new RuntimeException("Could not open the file!");
}
$FileRTF = new RTFFile();
$imgJPG = new RTFImage($sPathJPG);
if ($imgJPG->getHexImage() == "Error") {
throw new RuntimeException("Error: File \"".$sPathJPG."\" is not JPG or PNG");
}
/*
//predefined Colors that do not need to be added
//Name,Red,Green,Blue
$FileRTF->addColor("Negro",0,0,0); //Black ->Index(0)
$FileRTF->addColor("Azul",0,0,255); //Blue ->Index(1)
$FileRTF->addColor("Cian",0,255,255); //Cyan ->Index(2)
$FileRTF->addColor("Verde",0,255,0); //Green ->Index(3)
$FileRTF->addColor("Magenta",255,0,255); //Magenta ->Index(4)
$FileRTF->addColor("Rojo",255,0,0); //Red ->Index(5)
$FileRTF->addColor("Amarillo",255,255,0); //Yellow ->Index(6)
$FileRTF->addColor("Blanco",255,255,255); //White ->Index(7)
//predefined Fonts that do not need to be added
//Name,Family,FamilyName
$FileRTF->addFont("TlwgMono","fnil","TlwgMono");
$FileRTF->addFont("Arial","fswiss","Arial");
$FileRTF->addFont("TimesNewRoman","froman","Times New Roman");
$FileRTF->addFont("CourierNew","fmodern","Courier New");
$FileRTF->addFont("Edwardian","fscript","Edwardian Script ITC");
*/
$InfImagenJPG = $imgJPG->getRTFImage($imgJPG->getHexImage(),$imgJPG->getFormat(),
$imgJPG->getHigh(),$imgJPG->getWide());
$imgPNG = new RTFImage($sPathPNG);
if ($imgPNG->getHexImage() == "Error") {
throw new RuntimeException("Error: File \"".$sPathPNG."\" is not JPG or PNG");
}
$InfImagenPNG = $imgPNG->getRTFImage($imgPNG->getHexImage(),$imgPNG->getFormat(),
$imgPNG->getHigh(),$imgPNG->getWide());
$ImageRTFIMG = NULL;
$InfImagenIMG = NULL;
$PE = $newConn->prepare("SELECT * FROM tblRecords");
if (!$PE) {
throw new RuntimeException("Prepare SQL statement for creation failed!");
}
if (!$PE->execute()) {
throw new RuntimeException("Prepare SQL statement for execution failed!");
}
$PE->bind_result($sName,$sSalary,$sJoinDate,$BytesFoto,$BytesResume);
$PE->store_result();
$NumRecords = $PE->num_rows;
$TblData = NULL;
$FileRTF->addList("Lst1",RTFFile::LIST_UPPERROMANS,"1"); //Name,Type,FamilyFont#
$FileRTF->addListItem("Lst1","Primero");
$FileRTF->addListItem("Lst1","Segundo");
$FileRTF->addList("Lst2",RTFFile::LIST_LOWERALPHABET,"2");
$FileRTF->addListItem("Lst2","First");
$FileRTF->addListItem("Lst2","Second");
while ($PE->fetch()) {
$FileRTF->offOtherFormatFile();
$FileRTF->setAlign(RTFFile::ALIGN_CENTERED);
$FileRTF->setColorFront("Blanco");
$FileRTF->setColorBack("Negro");
$FileRTF->setFont("Edwardian");
$FileRTF->setSizeFont(20);
$FileRTF->setUnderLine(RTFFile::ON);
$FileRTF->addText("Limited Company");
$FileRTF->addParg();
$FileRTF->setColorBack("Blanco");
$FileRTF->setColorFront("Negro");
$TblData = new RTFTable(4,4); //4 Columns, 4 Rows
$TblData->setTableSpaceCellsRow(10);
$TblData->setTableLeftmostEdgePos(10);
$TblData->setTableHorzAlign(RTFTable::TABLE_LEFT);
$TblData->setTableBorderType(RTFTable::BORDER_SINGLETHICKNESS);
$TblData->setCellsVertAlign(RTFTable::CELLS_TOP);
$TblData->setCellsBorderType(RTFTable::BORDER_DOUBLETHICKNESS);
$TblData->setCellsThick(10);
$TblData->setCellsColor($FileRTF->getColorIndex("Negro"));
$TblData->setWideCols(1000); //Width of 2000 to put all columns
$TblData->setWideCol(0,3000); //Width of 3000 to Column 0
$TblData->setWideCol(3,3000); //Width of 3000 to Column 3
$TblData->setAlignTable(RTFTable::ALIGN_CENTERED);
$TblData->setColorFrontTable($FileRTF->getColorIndex("Blanco"));
$TblData->setColorBackTable($FileRTF->getColorIndex("Negro"));
$TblData->setFontTable($FileRTF->getFontIndex("TlwgMono"));
$TblData->setSizeFontTable(12);
$TblData->setAlignCell(0,0,RTFTable::ALIGN_LEFT); //Col,Row,Align
$TblData->setColorFrontCell(0,0,0); //Col,Row,ColorIndex
$TblData->setColorBackCell(0,0,0); //Col,Row,ColorIndex
$TblData->setFontCell(0,0,0); //Col,Row,Font
$TblData->setSizeFontCell(0,0,12); //Col,Row,Size
$TblData->setItemCell(0,1,$InfImagenPNG);
$TblData->setItemCell(3,1,$InfImagenJPG);
$TblData->mergeHorzCell(1,0,2); //Col,Row,NumCellToBeMergedHorizontally
$TblData->setTextCell(1,0,"Celda 1,0 con Celda 2,0");
$TblData->mergeHorzCell(1,3,2);
$TblData->setTextCell(1,3,"Celda 1,3 con Celda 2,3");
$TblData->mergeVertCell(0,1,2); //Col,Row,NumCellToBeMergedVerticaally
$TblData->mergeVertCell(3,1,2);
$FileRTF->addItem($TblData->getTable());
$FileRTF->addLine();
$FileRTF->setUnderLine(RTFFile::OFF);
$FileRTF->setSizeFont(11);
$FileRTF->setFont("CourierNew");
$FileRTF->setScript(RTFFile::SUB);
$FileRTF->addText("SUBSCRIPT");
$FileRTF->setScript(RTFFile::OFF);
$FileRTF->addText(" ");
$FileRTF->setStrike(RTFFile::ON);
$FileRTF->addText("STRIKE");
$FileRTF->setStrike(RTFFile::OFF);
$FileRTF->addText(" ");
$FileRTF->setScript(RTFFile::SUPER);
$FileRTF->addText("SUPERSCRIPT");
$FileRTF->setScript(RTFFile::OFF);
$FileRTF->addLine();
$FileRTF->setAlign(RTFFile::ALIGN_LEFT);
$FileRTF->setFont("CourierNew");
$FileRTF->setSizeFont(12);
$FileRTF->setUnderLine(RTFFile::ON);
$FileRTF->setBold(RTFFile::OFF);
$FileRTF->setItalic(RTFFile::OFF);
$FileRTF->setColorFront("Negro");
$FileRTF->addText("Nombre: ");
$FileRTF->setItalic(RTFFile::ON);
$FileRTF->setUnderLine(RTFFile::OFF);
$FileRTF->setColorFront("Rojo");
$FileRTF->addText(utf8_decode($sName));
$FileRTF->addLine();
$FileRTF->setItalic(RTFFile::OFF);
$FileRTF->setUnderLine(RTFFile::ON);
$FileRTF->setColorFront("Negro");
$FileRTF->addText("Salario: ");
$FileRTF->setItalic(RTFFile::ON);
$FileRTF->setUnderLine(RTFFile::OFF);
$FileRTF->setColorFront("Rojo");
$FileRTF->addText($sSalary);
$FileRTF->addLine();
$ImageRTFIMG = new RTFImage($BytesFoto);
/*
//$ImageRTFIMG->setCropLeft(0);// 0 cutted twips in Left Side
//$ImageRTFIMG->setCropRight(0);// 0 cutted twips in Right Side
//$ImageRTFIMG->setCropTop(0);// 0 cutted twips in Top Side
//$ImageRTFIMG->setCropBottom(0);// 0 cutted twips in Bootom Side
//$ImageRTFIMG->setScaleX(100);// 100% of X Image
//$ImageRTFIMG->setScaleY(100);// 100% of Y Image
//*/
$ImageRTFIMG->setScaleX(50);
$ImageRTFIMG->setScaleY(25);
$InfImagenIMG = $ImageRTFIMG->getRTFImage($ImageRTFIMG->getHexImage(),
$ImageRTFIMG->getFormat(),$ImageRTFIMG->getHigh(),$ImageRTFIMG->getWide());
$FileRTF->addItem($InfImagenIMG);
$FileRTF->addParg(); //Separe Image of Text
$FileRTF->setItalic(RTFFile::OFF);
$FileRTF->setUnderLine(RTFFile::ON);
$FileRTF->setColorFront("Negro");
$FileRTF->addText("Resumen: ");
$FileRTF->setItalic(RTFFile::ON);
$FileRTF->setUnderLine(RTFFile::OFF);
$FileRTF->setColorFront("Verde");
$FileRTF->addText(utf8_decode($BytesResume));
$FileRTF->setColorFront("Azul");
$FileRTF->addLine();
$FileRTF->addText("Ini List");
$FileRTF->setFirstIndent(-360);
$FileRTF->setLeftIndent(720);
$FileRTF->setSpaceAfter(RTFFile::ON);
$FileRTF->setSpaceMultiple(RTFFile::ON);
$FileRTF->addItem($FileRTF->getList("Lst1"));
$FileRTF->offIndent();
$FileRTF->offSpacing();
$FileRTF->incIndent();
$FileRTF->incSpacing();
$FileRTF->addLine();
$FileRTF->addText("Mid List");
$FileRTF->setSpaceLine(RTFFile::SL_2_0); //Line Spacing 2.0
$FileRTF->setRightIndent(1040);
$FileRTF->setSpaceBefore(RTFFile::ON);
$FileRTF->setSpaceMultiple(RTFFile::ON);
$FileRTF->addItem($FileRTF->getList("Lst2"));
$FileRTF->offIndent();
$FileRTF->offSpacing();
$FileRTF->incIndent();
$FileRTF->incSpacing();
$FileRTF->setColorFront("Negro");
$FileRTF->addText("End List");
$FileRTF->addLine();
$FileRTF->addText("Record: ".$NumRecords);
$FileRTF->addLine();
$FileRTF->addText("Last Text");
$NumRecords--;
if ($NumRecords>0) {
$FileRTF->addParg();
$FileRTF->addPage(); //New Page
}
}
$FileRTF->endFile();
fwrite($fw,$FileRTF->getFile());
fclose($fw);
printLnk($WebFileName);
printMsg("Successful generation of RTF file!");
}
catch (Exception $e) { printErr($e->getMessage()); }
finally {
$PE->close();
$newConn->close();
}
}
//Elimination
if (getRequestStr("bDelt")!= NULL) {
try {
$PE = $newConn->prepare("DELETE FROM tblRecords");
if (!$PE) {
throw new RuntimeException("Prepare SQL statement for creation failed!");
}
if (!$PE->execute()) {
throw new RuntimeException("Prepare SQL statement for execution failed!");
}
printMsg("Successful elimination!");
}
catch (Exception $e) { printErr($e->getMessage()); }
finally {
$PE->close();
$newConn->close();
}
}
}
catch (Exception $e) { printErr($e->getMessage()); }
?>
«dbUserf», «dbKeyf» and «dbCompanyf» are values assigned incorrectly (on purpose) to test session functions instead of «dbUser», «dbKey» and «dbCompany».
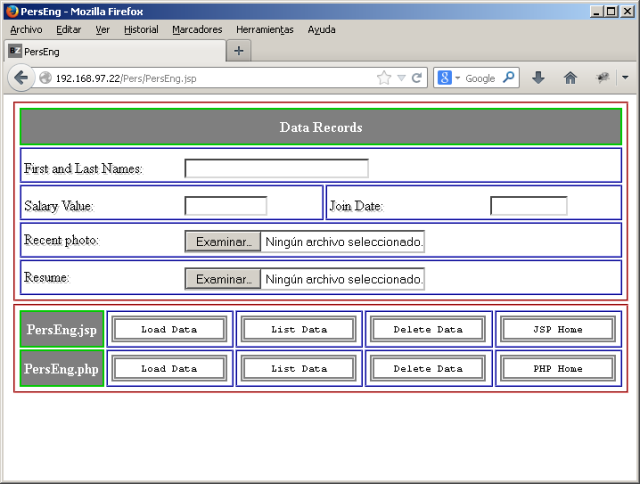
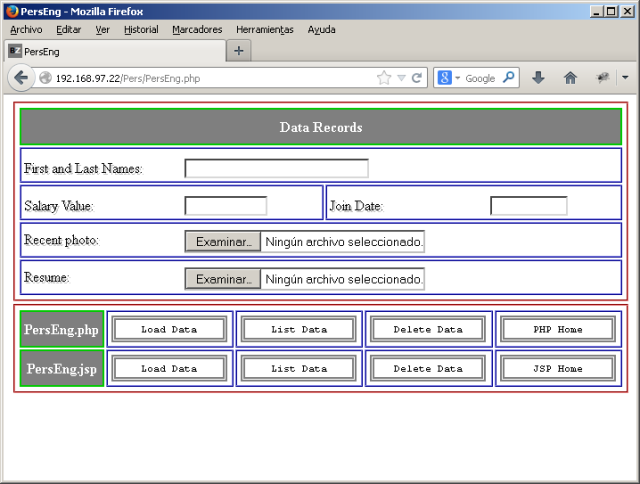
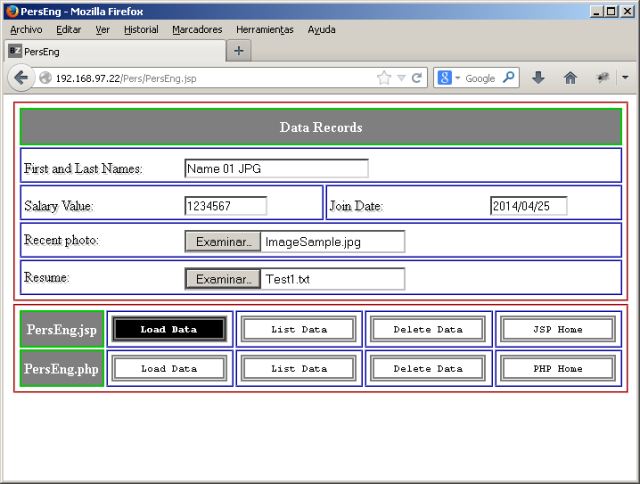
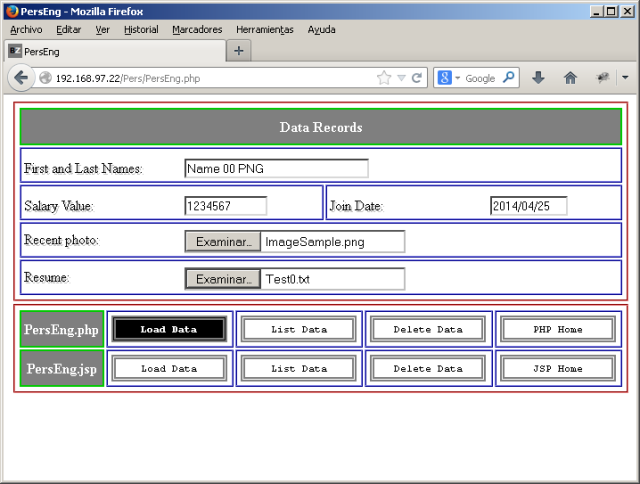
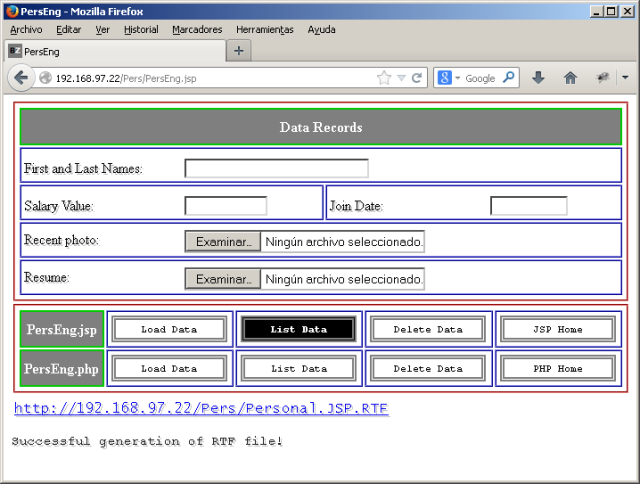
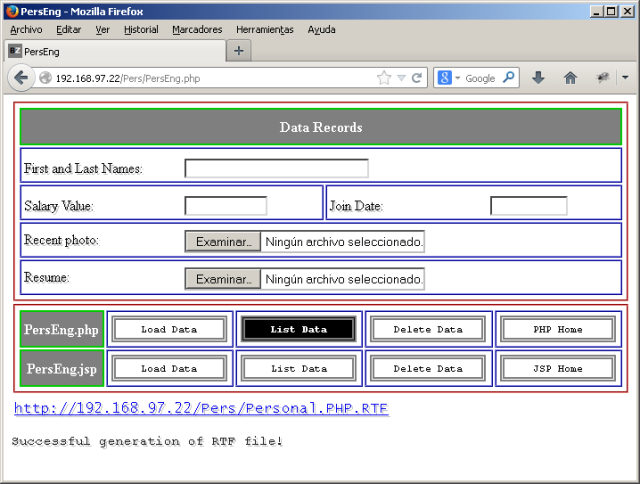
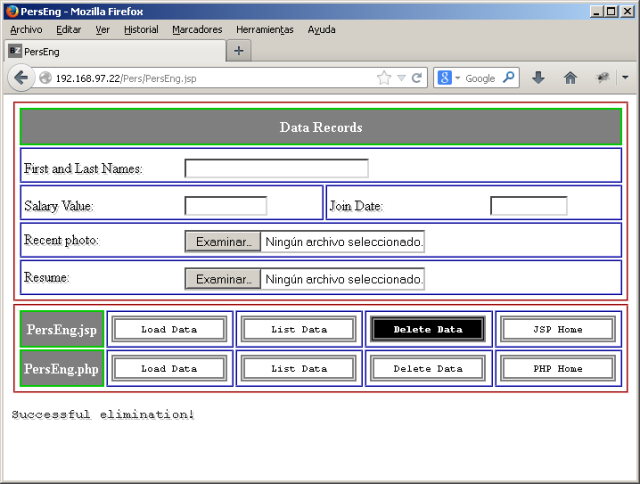
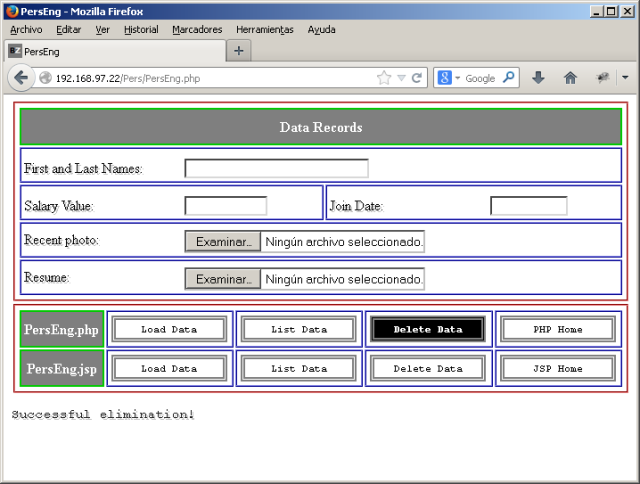
The result of this test is the RTF file:
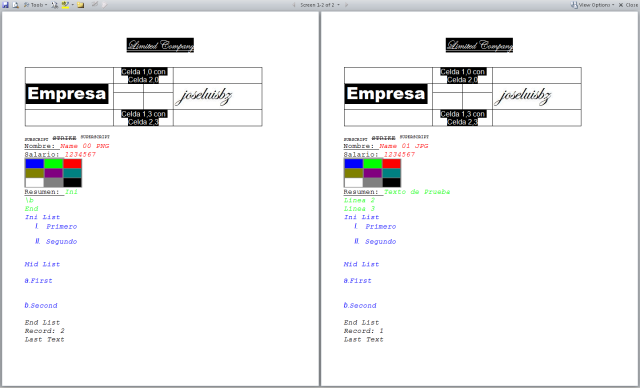
Comments:
If you have problem, comments or questions using this post, let me know.